In early December last year we replaced the unspeakably disgusting carpet in the loungeroom (it had been there since the house was built in the mid-80s) with some rather nice tiles. Fallout from that process involved getting rid of our Ikea Billy cd shelves, moving a bookcase from one wall to another, and EOLing (end-of-life) our Beyonwiz DPP2 pvr. We haven't recorded live tv in a ~very~ long time, so the DPP2 was a rather expensive way of providing an ntp-anchored clock.
J expressed a desire for a replacement clock, and I've always appreciated having an actually accurate clock. So I acquired an Adafruit PiTFT panel. After being surprised that I had to solder the 40pin socket connector myself (not having soldered anything in more than 10 years), I managed to do it sufficiently well that the device was fine on boot and got a working display:
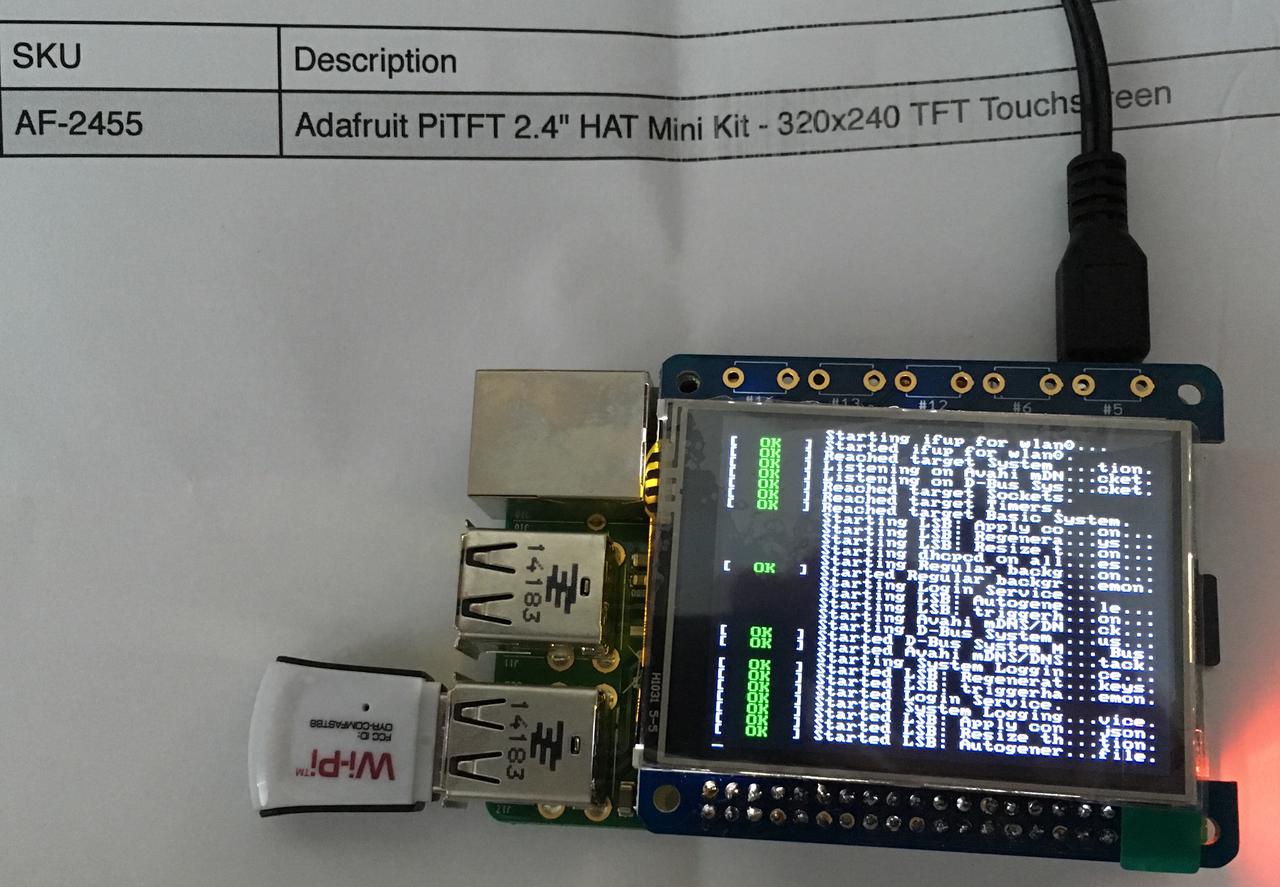
Now since the Pi in the loungeroom runs OSMC, that is, it's an appliance, it doesn't have the requisite Adafruit drivers in its repo. So... time to build a fresh kernel.
I build fresh Solaris kernels several times a day, and in 2014 Tim, Mark and I delivered a major rewrite for how we actually build core Solaris. But I haven't built a linux kernel in about 20 years - I had to go looking for instructions on where to start! I've taken my lead from *khAttAm* and now I've got the repo building on the pi. It's going to take a while, though, because (a) the pi is fairly low-powered, and (b) I've set it up so that the OSMC home directory is actually mounted from our Solaris media server so we don't run out of space with the media db.
Anyway, once that kernel and its modules are built, I hope to schlep them into
place, suddenly have a /dev/fb1
on which to display this:
#!/usr/bin/python3.4 # from http://stackoverflow.com/questions/7573031/when-i-use-update-with-tkinter-my-label-writes-another-line-instead-of-rewriti # only slightly modified import tkinter as tk import time class piClocknDate(tk.Tk): def __init__(self, *args, **kwargs): tk.Tk.__init__(self, *args, **kwargs) self.maxsize(width=320, height=240) self.resizable(0, 0) self.title("rpi Clock") self.fontC = "helvetica 36 bold" self.fontD = "helvetica 18 bold" self.padc = 40 self.padd = 50 self.clockL = tk.Label(self, text="", font=self.fontC, padx=self.padc, pady=70, foreground="light blue", background="black") self.clockL.pack() self.curdate = time.strftime("%d %B %Y", time.localtime()) self.dateL = tk.Label(self, text=self.curdate, font=self.fontD, padx=self.padd, pady=70, foreground="blue", background="black") self.dateL.pack() # start the clock "ticking" self.update_clock() self.update_date() def update_clock(self): curt = time.localtime() disptime = time.strftime("%I:%M %p" , curt) secs = int(time.strftime("%S")) padx = self.padc if secs % 15 is 0: padx = self.padc - 10 self.clockL.configure(text=disptime, padx=padx) # call this function again in one second self.after(1000, self.update_clock) def update_date(self): newdate = time.strftime("%d %B %Y", time.localtime()) if newdate is not self.curdate: self.curdate = newdate self.dateL.configure(text=self.curdate, padx=self.padd) self.after(1000, self.update_date) if __name__== "__main__": app = piClocknDate() app.mainloop()